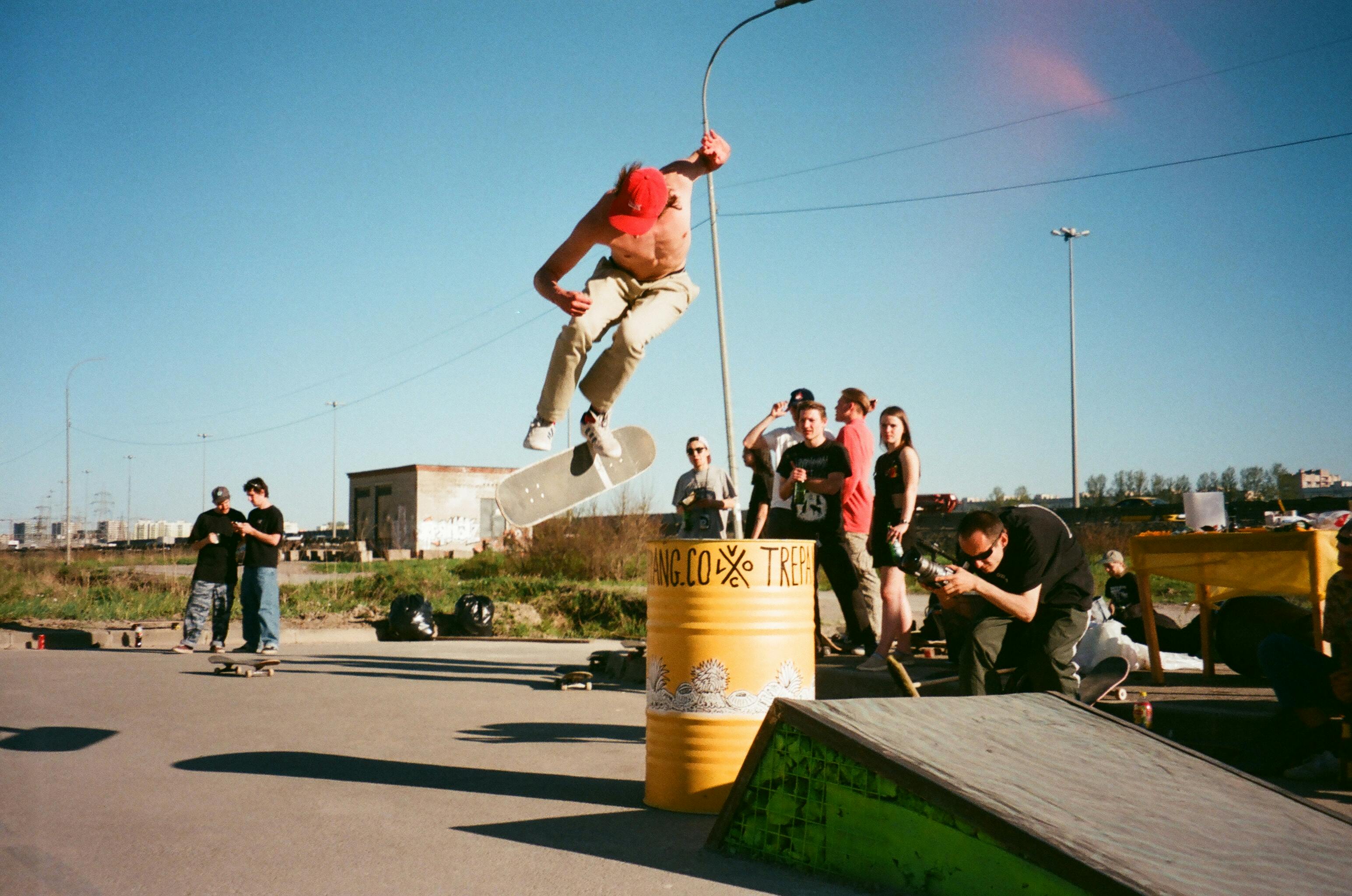
Fluid Animation in C#
Generating smooth animations with C# on different computer systems can be a challenging task. However, there is a simple method for adjusting a program’s behavior to a target number of frames per second.
Animating objects with C# can mean a number of different things.
The first would be to animate a sequence of images as an animated GIF. In this case, there is already some support in the .Net Framework, but you may want to handle frame-by-frame drawing.
Another form of animation is with images generated by code. GDI%2B can draw some very complex graphics and animating them smoothly can add an extra dimension of quality to your .Net applications.
Finally, sometimes programmers want to animate Window Form behavior such as size, position, text, etc. While these are object properties, we want them to change at a regular interval, regardless of the computer’s CPU speed.
The first step to writing constant FPS animations in C# is deciding how to measure CPU cycles. The most commonly used objects for measuring time are the Timer object and the DateTime class. While both are easy to use, they lack precision. Instead, we’re going to make use of the System.Diagonistics.StopWatch class.
The StopWatch class uses the QueryPerformanceTimer API call to keep track of CPU cycles, which is more accurate. Higher precision in this case means more consistent animation.
Basically, you’ll want to keep track of three values, two of which will be constantly changing:
- Interval = Stopwatch.Frequency/ [target FPS (30.0 for example)]
- currentTicks = Stopwatch.GetTimestamp()
- lastTicks = same as currentTicks but taken last time animation was drawn
The logic behind the C# algorithm is not overly complicated. Simply put, a loop will run constantly, but you only want the animation to run/update when the last number of CPU cycles and the current number of cycles are at least one gap apart from the Interval you previously calculated. Then, and only then, is the animation updated.
The result is constant animation no matter how fast a computer is. Just adjust the FPS on your own system and that will be the perceived speed on all systems.
The reason it works is because the animations don’t run in a simple brainless while/for loop. The loop uses the host computer’s CPU cycles to adjust the interval at which the animation is updated.